- Published on
JavaScript Intermediate Part- 2
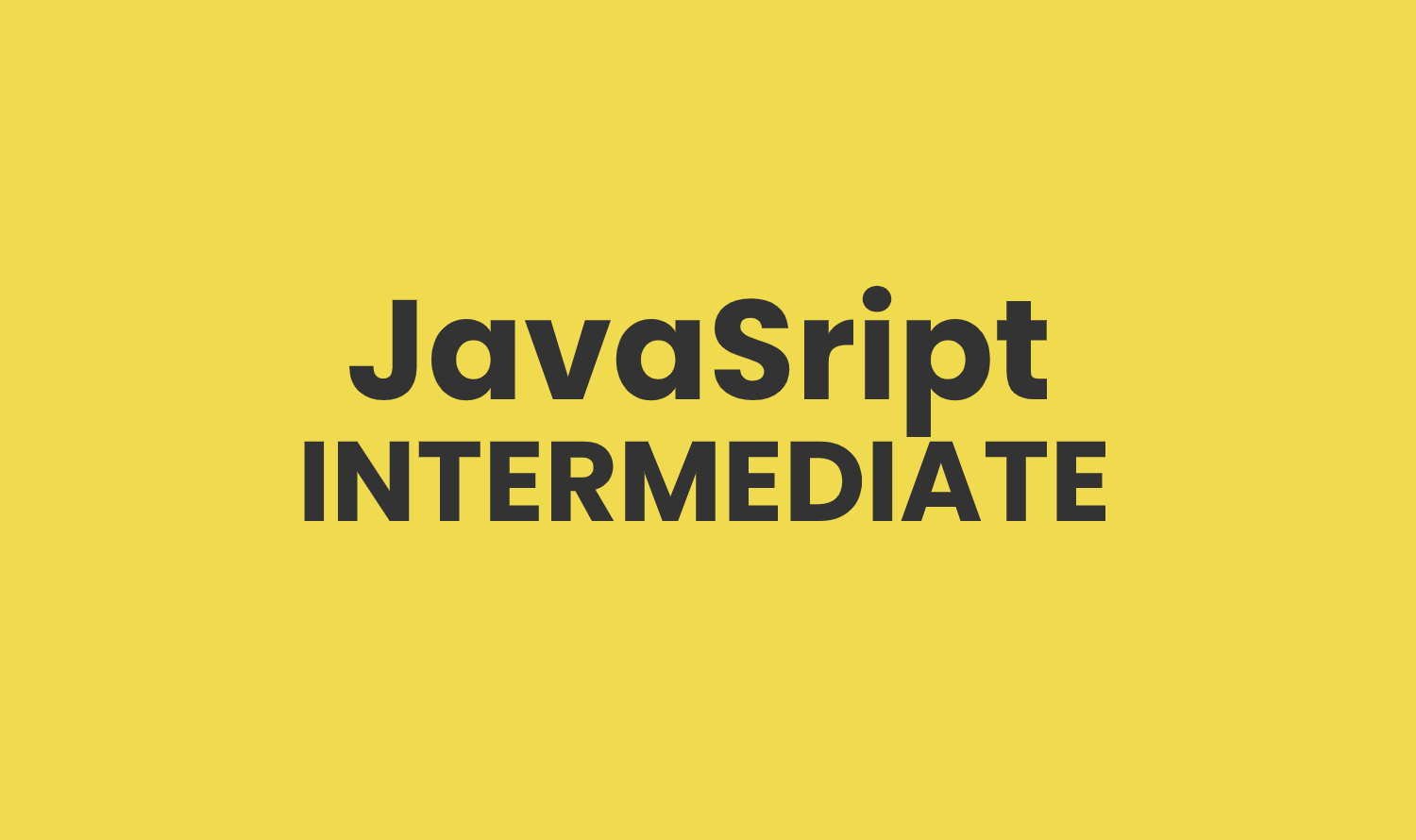
#Related Topics:
This articles covers these topics api, json, json parse, json stringify, load data, get data, display data on UI, http request status code, network tab, send data to server, object method, bind, call, apply, window, global scope, new keyword, class, this keyword, async, await, setTimeout, setInterval, event loop and datetime
1 API JSON, SERVER, DATA LOAD, DYNAMIC WEBSITE, HTTP
1.1 WHAT IS AN API, THE PURPOSE OF API, GET, POST
1.2 JSON, JSON STRUCTURE, PARSE, STRINGIFY, JSON PROPERTIES
html
1.3 LOAD DATA, JSON PLACEHOLDER, GET DATA, DISPLAY DATA ON UI
html
1.4 HTTP REQUEST, STATUS CODE, NETWORK TAB, BAD API
html
1.5 SEND DATA TO THE SERVER, HTTP POST METHOD, GET VS POST AND SEND DATA TO SERVER, HTTP POST, JSON STRINGIFY
html
Additional Resources - 1
Additional Resources - 2
1.6 JQUERY AJAX, JQUERY GET, JQUERY POST REACT INSTALL ISSUE
2 OBJECT MASTERING, INTERVIEW QUESTION
2.1 OBJECT METHOD PROPERTY REVIEW
javascript
2.2 OBJECT USE BIND TO BORROW METHOD FROM ANOTHER OBJECT
javascript
Additional Resources - 1
Additional Resources - 2
2.3 DIFFERENCE BETWEEN BIND, CALL AND APPLY
javascript
Additional Resources - 1
Additional Resources - 2
Additional Resources - 3
Additional Resources - 4
2.4 WINDOW, GLOBAL VARIABLE, GLOBAL SCOPE
html
2.5 NEW KEYWORD, CLASS AND OBJECT DIFFERENCE
javascript
2.6 HOW TO UNDERSTAND THE THIS KEYWORD
html
2.7 ASYNC AWAIT HOW TO USE IT FOR ASYNC CALL
html
Additional Resources - 1
Additional Resources - 2
Additional Resources - 3
Additional Resources - 4
2.8 ASYNCHRONOUS JAVASCRIPT SETTIMEOUT, SETINTERVAL
javascript
2.9 HOW JAVASCRIPT WORKS EVENT LOOP STACK AND QUEUE
2.10 JAVASCRIPT DATETIME TIMEZONE AND OTHERS
javascript