- Published on
JavaScript Basics Part- 2
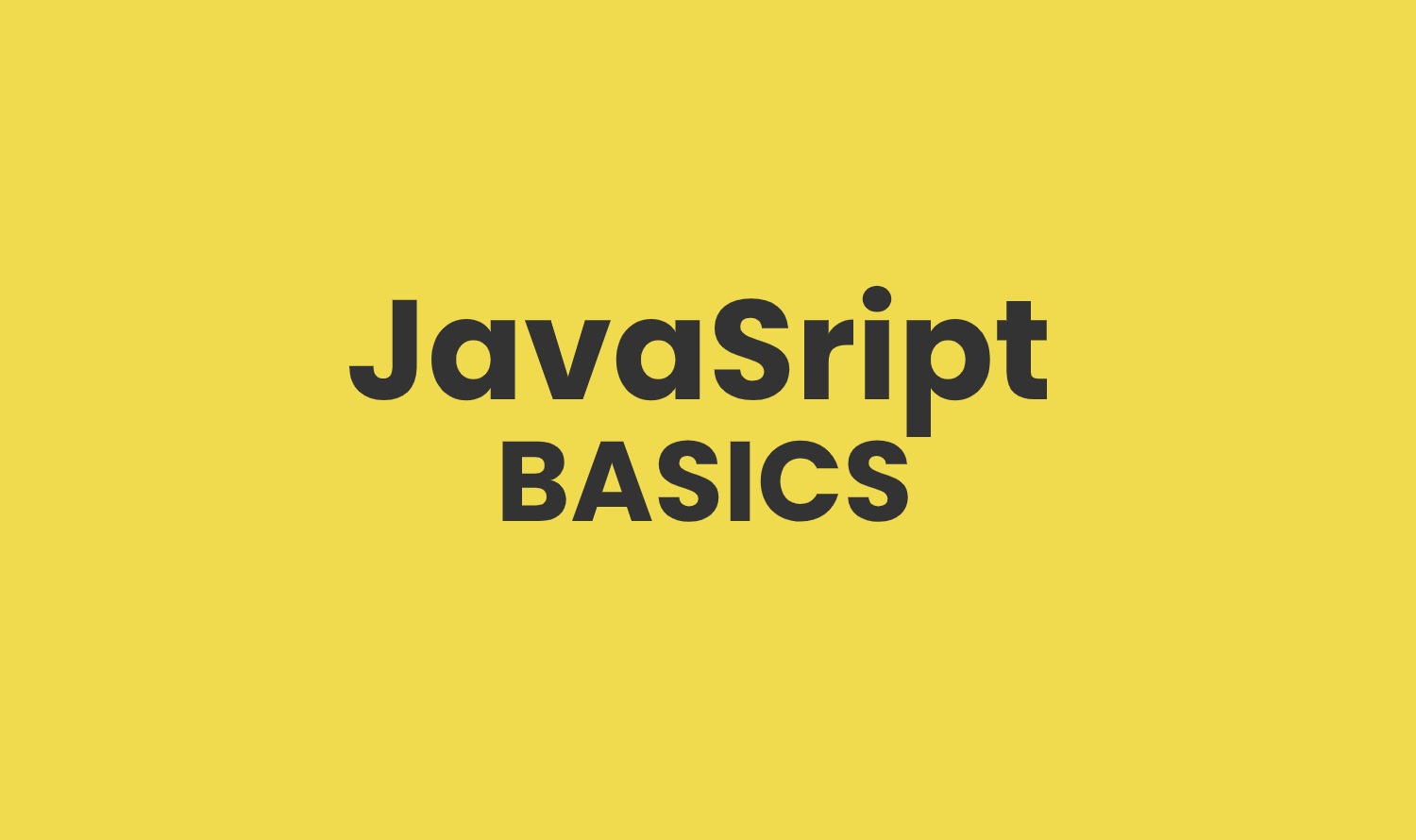
#Related Topics:
1 APPLY JAVASCRIPT CONCEPTS
1.1 UNIT CONVERT INCH TO FEET USE VARIABLE AND FUNCTION
javascript
1.2 VARIABLE LET AND CONST AND HOW TO USE THEM
javascript
1.3 CHECK WHETHER A YEAR IS A LEAP YEAR OR NOT
javascript
Correct way of finding leap year:
To determine whether a year is a leap year, follow these steps:
- If the year is evenly divisible by 4, go to step 2. Otherwise, go to step 5.
- If the year is evenly divisible by 100, go to step 3. Otherwise, go to step 4.
- If the year is evenly divisible by 400, go to step 4. Otherwise, go to step 5.
- The year is a leap year (it has 366 days).
- The year is not a leap year (it has 365 days).
or
- If a year is divisible by 400, it's a leap year
- Otherwise, if a year is divisible by 100, it's not a leap year
- Otherwise, if a year is divisible by 4, it's a leap year
javascript
1.4 CALCULATE FACTORIAL OF A NUMBER USING FOR LOOP
javascript
1.5 CALCULATE FACTORIAL OF A NUMBER USING A WHILE LOOP
javascript
1.6 CALCULATE FACTORIAL IN A RECURSIVE FUNCTION
javascript
1.7 CREATE A FIBONACCI SERIES USING A FOR LOOP
[About Fibonacci Series](https:// www.mathsisfun.com/numbers/fibonacci-sequence.html)
javascript
1.8 FIBONACCI ELEMENT IN A RECURSIVE WAY
javascript
1.9 CREATE FIBONACCI SERIES IN A RECURSIVE WAY
javascript
1.10 CHECK WHETHER A NUMBER IS A PRIME NUMBER OR NOT
javascript
2 JAVASCRIPT CODING PROBLEMS, SIMPLE INTERVIEW QUESTIONS
2.1 SWAP VARIABLE, SWAP WITHOUT TEMP, DESTRUCTING
javascript
2.2 RANDOM NUMBER, RANDOM NUMBER BETWEEN 1 TO 6
javascript
2.3 FIND MAX OF TWO VALUES, FIND MAX OF THREE VALUES
javascript
2.4 FIND THE LARGEST ELEMENT OF AN ARRAY
javascript
2.5 SUM OF ALL NUMBERS IN AN ARRAY
javascript
2.6 REMOVE DUPLICATE ITEM FROM AN ARRAY
javascript
2.7 COUNT THE NUMBER OF WORDS IN A STRING
javascript
2.8 REVERSE A STRING
javascript
3 Exercise
3.1 FIND THE LARGEST VALUE
javascript
3.2 WRITE TWO FUNCTIONS TO FIND FACTORIAL
javascript
3.3 FIND THE NUMBER OF ANIMAL
javascript