- Published on
10 fundamental things you need to know about React
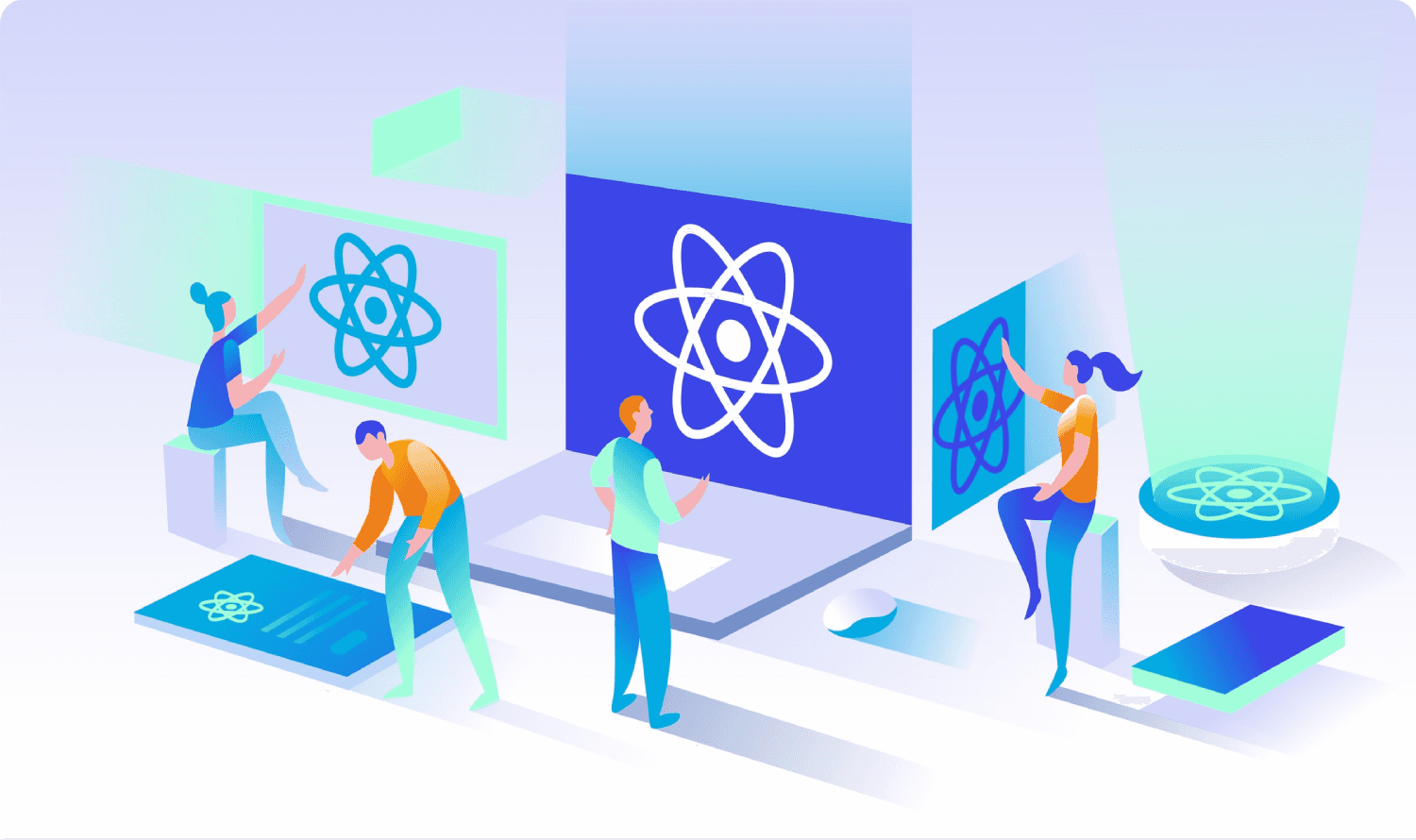
Before begin I want to let you know this is not the complete guide of react fundamental rather this is introductory guide of react fundamental concepts. I will discuss 10 fundamental concepts about react that you must know as a react developer.
1. What is React anyways?
React is a JavaScript 'library' not a 'framework' developed by Facebook . With framework like angular, vue the control is inverted means framework will made decision for you, whereas with library like react you can make your own decision, you are in control. React helps builds user interfaces for single-page applications (SPA) by dividing UI reusable React library code (saving development time and reduce the coding errors). You
2. JSX (JavaScript Extension)
JSX is an syntax extension to JavaScript that allows to write function calls in an HTML-like syntax. Though JSX look like html, it's no html and it's not understood by browser. JSX is basically a compromise between react and browser. React uses babel transpiler to translate JSX syntax to react component like React.createElement
. Consider this example:
Behind the scenes, each element rendered by the Greeting component is transpiled by babel into to a React.createElement.
This is also true for nested elements. For example:
3. How JavaScript work in React
We can use JS in react, consider an array of objects with property id and name. We can use ``map` function to show it in the DOM like that.
4. React is declarative
React use declarative approach, with react you can build user interfaces without even touching the DOM directly, not only that, you can implement event system without interactive with actual DOM events. We just tell React we want a component to be rendered in a specific way. React will take care of the how to do it and translate our declarative abstract to actual UIs in the browser and if any data change react will efficiently update and render the right component.
5. Reusable Components
React provides component based structure written in JavaScript. Component is like functions, when we need it, we call it, with some given input we get some output. Components are reusable, composable, and stateful. React use concept of 'props' as input while you need to pass data in component children in order for the children to render properly. A React component can be either 'stateful' or 'stateless'. Class components are 'Stateful' where as function components are 'stateless'.
6. React Hooks
React hooks were introduced in React 16.8. React hook make it easier to use state and react life cycle feature using function component and without using class
and react component life cycle method. React hooks are special type of function allow you to add state like useState
hook, and useEffect
hook allows you to perform side effect. Before, side effects were implemented using react component life cycle method. useState
hook help to track state update within a component and trigger the virtual DOM diffing. useState
hook can be declared in component as follows:
The useState
hook returns an array with exactly 2 items. The first item is a value and the second item is a function. The first item “value” can be a string, number, array, or other types. In the above code I used to 0 as initialize value, The second item 'function' will change the value of the state when the setCount
is invoked and update the DOM.
7. Data Flow
In React data flow allows us to send data between parent and child components as props but components that are siblings cannot directly communicate with each other. Another way of data flow is to use Redux or some stage management library. Example of parent child component data flow:
8. Conditional Rendering
It's often required to render some parts or component if certain condition is met and other is not met. There are lot's of different ways we can apply conditional rendering in React. For example, these piece of conditional rendering can change the button label based on user logged in or not.
9. Default Props
Consider this React component.
The above code defines a very simple Header component that renders a <h1>
element containing a heading. What happens if title prop is not passed?
If the title prop is not passed, the reference to props.title will be undefined.
One way to resolve this issue is to use conditional rendering:
Another way to resolve this issue is to set a default props for the component or fallback value.
10. Fast render with Virtual DOM
Refreshing DOM is generally the bottleneck with regards to the web application performance. React is attempting to take care of this issue by utilizing something many refer to as virtual DOM; a DOM kept in memory. Any view changes are first reflected to virtual DOM, at that point a proficient diff calculation looks at the past and present status of the virtual DOM and figures the most ideal way to apply these changes. At last only those updates are applied to the DOM only at the node where there is an actual change along with it’s children. This is the main reason behind React's performance.