- Published on
JavaScript frequently asked problem solving question with solution
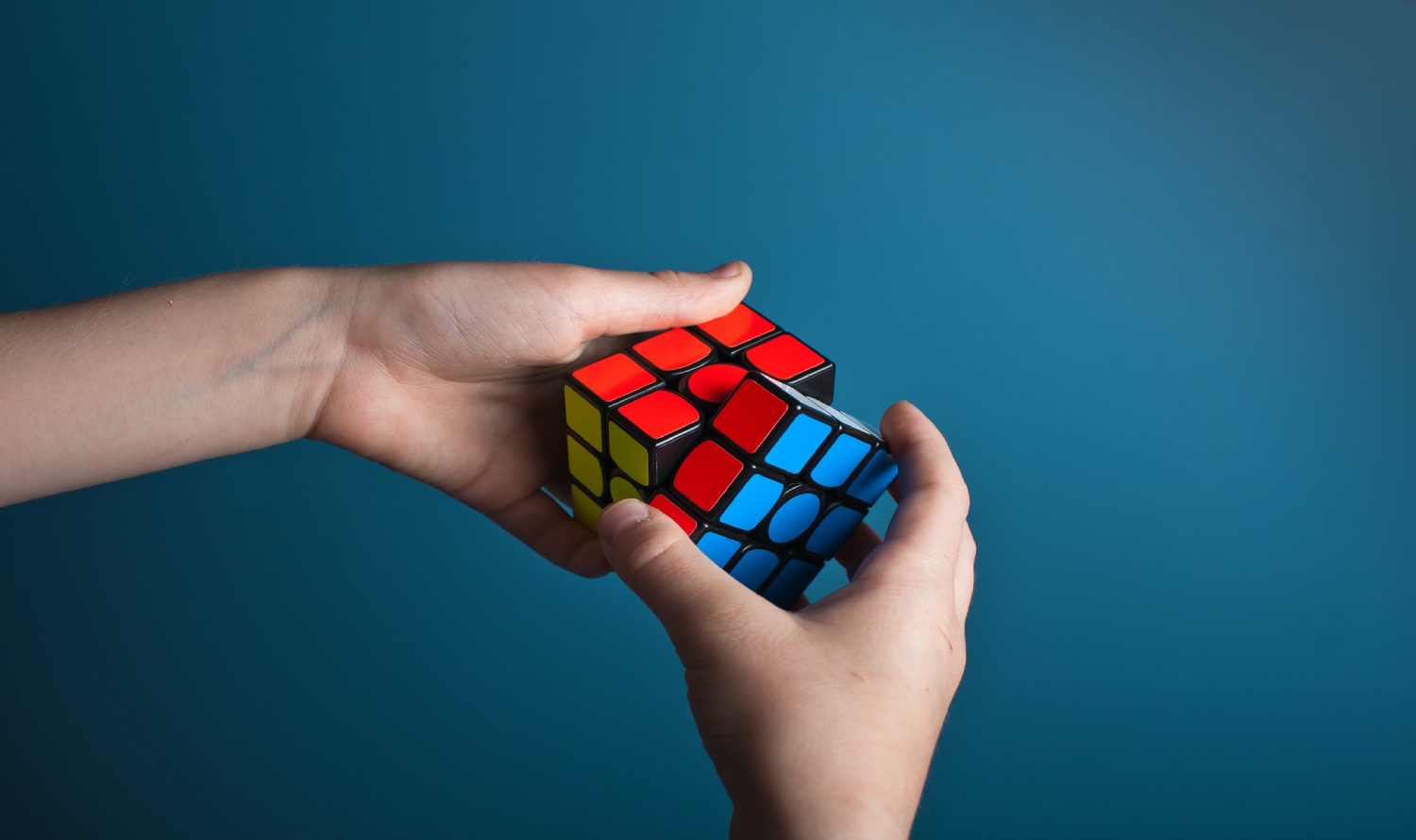
1. Find The Largest Element Of An Array
There are multiple ways to find out the largest value of an array. One way is to use Math.max()
function to find the largest value.
But often interviewer may ask you to write a for loop to find the largest value of an array. Let's do the same exercise with for loop.
Also, we can write a reusable function to find the largest element of an array.
2. Find Sum Of All Numbers In An Array
Like finding the largest number, there are multiple ways to find the sum of all numbers in an array.
We can calculate the sum using for loop:
We can write a function as well:
Also another way to find the sum is to use reduce
higher order function:
3. Remove Duplicate Item From An Array
It is often require to deal with duplicate number, we can remove duplicate from an array in multiple ways.
We can remove duplicates using for loop:
We can write a function like this also:
Remove duplicate using indexOf
and filter
:
Remove duplicate using includes
and forEach
:
4. Count The Number Of Words In A String
There are lots of ways to count the number of words in a string like using for loop, using regex with string method like replace, split etc.
Let's solve the problem with for loop first, then I will share a simple solution.
Also we can create a simple function like this to find the number of words:
5. Reverse A String
This is one of the common javascript problem solving question. There are multiple ways to solve this problem, I will share two solution, one using for loop and another with string method like split, reverse and join.
Using for loop:
Using string method:
6. Check Whether A Year Is A Leap Year Or Not
There are multiple ways we can find a year is a leap year or not. But first we need to understand the logic, when we call a year as leap. There are few conditions you will need to check if you want to identify leap year. These conditions are:
To determine whether a year is a leap year, follow these steps:
- Step 1. Divide a year by 400, if it's divisible then it's a leap year. If not divisible follow step 2.
- Step 2. Divide a year by 100, if it's divisible then it's not a leap year. If divisible follow step 3.
- Step 3. Divide a year by 4, if it's divisible then it's a leap year.
We can implement these above condition in various way:
7. Calculate Factorial Of A Number Using For Loop
Before calculating factorial first we need to understand how factorial calculation works. In mathematics we use exclamation to denote factorial like 5! (five factorial). What that means is nothing but the product of positive integer from 1 to n (1 to 5 in this case) like (54321). So 5! is equivalent to 120. We can implement factorial calculation in multiple ways. I will share how we can use for loop to calculate factorial then will share how we can create a function for calculating factorial.
8. Calculate Factorial Of A Number Using A While Loop
In the above problem solving exercise we used for loop, similarly we can use while loop for factorial calculation in following way:
9. Calculate Factorial In A Recursive Function
Another way to find the factorial is using recursive function. What is recursive function anyways? Recursive means repetition, when a function call itself and repeat the process until certain condition is met, then it's called recursive function. To find the factorial in recursive way, first we need to understand what other alternative way we can write factorial, in a way that we can repeat the process until a certain condition met. Since 5! is equivalent to 4!*5
, which is equivalent to (5-1)!*5
, for a whole number n we can write (n-1)!*n
. We will use similar technique in recursive function so that we can repeat the process.
In the following function when function calls itself and after each iteration the number n will change and when n get to 0 the function will stop iteration.
10. Create A Fibonacci Series Using A For Loop
A fibonacci sequence is a series of numbers: 0, 1, 1, 2, 3, 5, 8, 13, 21
To create fibonacci series first we need to understand how each number in fibonacci sequence calculated. In fibonacci sequence any next number is calculated by adding two number before it. For example, in the above sequence the third number is calculated (0+1) or 1, fourth number is calculated (1+1) or 2 and so on. We need to implement the same logic in such a way that we can add two numbers for next number. In simple way we can write:
Now we can apply for loop to create fibonacci series:
11. Fibonacci Element In A Recursive Way
We already learned about recursive function. To find a fibonacci element we need to create and repeat the similar formula we used in the previous example using recursive function. Since in fibonacci sequence any next number is calculated by adding two number before it, and the sequence start with 0 , 1, we can create the following function to get a fibonacci element.
12. Create Fibonacci Series In A Recursive Way
Before I showed you how to use for loop to create fibonacci series, in this problem solving exercise I will use recursive function to create fibonacci series. In this recursive function we will repeat the addition process until n equal 0 or n equal 1, and push each element in each iteration.
13. Check Whether A Number Is A Prime Number Or Not
When an integer number is not a product of two other integer number we call that number as prime number. We can verify a number is prime or not in this following way: